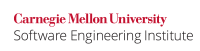
The varargs feature was introduced in the JDK v1.5.0 to support methods that accept variable numbers of arguments.
According to the Java SE 6 documentation [[Sun 2006]] varargs
As an API designer, you should use [varargs methods] sparingly, only when the benefit is truly compelling. Generally speaking, you should not overload a varargs method, or it will be difficult for programmers to figure out which overloading gets called.
Noncompliant Code Example
Overloading varargs methods creates confusion, as shown in this noncompliant code example. The programmer's intent is to invoke the variable argument (varargs) doSomething()
method, but instead its overloaded, more specific form takes precedence.
class OverloadedVarargs { private static void doSomething(boolean... bool) { System.out.print("Number of arguments: " + bool.length + ", Contents: "); for (boolean b : bool) System.out.print("[" + b + "]"); } private static void doSomething(boolean bool1, boolean bool2) { System.out.println("Overloaded method invoked"); } public static void main(String[] args) { doSomething(true, false); } }
Compliant Solution
Avoid overloading varargs methods. Use distinct method names to ensure that the intended method is invoked, as shown in this compliant solution.
class NotOverloadedVarargs { private static void doSomething1(boolean... bool) { System.out.print("Number of arguments: " + bool.length + ", Contents: "); for (boolean b : bool) System.out.print("[" + b + "]"); } private static void doSomething2(boolean bool1, boolean bool2) { System.out.println("Overloaded method invoked"); } public static void main(String[] args) { doSomething1(true, false); } }
Exceptions
DCL08-EX1: It may be desirable to violate the "Avoid overloading varargs methods" advice for performance reasons (avoiding the cost of creation of an array instance and its initialization on every invocation of a varargs method). [[Bloch 2008]]
public void foo() { } public void foo(int a1) { } public void foo(int a1, int a2, int... rest) { }
The idiom shown above avoids the pitfalls of incorrect method selection by using non-ambiguous method signatures; it may be used where required.
Risk Assessment
Unmindful use of the varargs feature may create ambiguity and diminish code readability.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
DCL08-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
Automated detection is straightforward.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Bibliography
[[Bloch 2008]] Item 42: "Use varargs judiciously"
[[Steinberg 2005]] "Using the Varargs Language Feature"
[[Sun 2006]] varargs
DCL07-J. Beware of integer literals beginning with '0' 03. Declarations and Initialization (DCL) DCL09-J. Enforce compile-time type checking of variable argument types