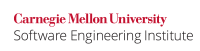
Division and remainder operations are susceptible to divide-by-zero errors. Consequently, the divisor in a division or remainder operation must be checked for zero prior to the operation.
Noncompliant Code Example (Division)
The result of the /
operator is the quotient from the division of the first arithmetic operand by the second arithmetic operand. Division operations are susceptible to divide-by-zero errors. Overflow can also occur during two's-complement signed integer division when the dividend is equal to the minimum (negative) value for the signed integer type and the divisor is equal to —1. See rule NUM00-J. Detect or prevent integer overflow for more information. This noncompliant code example can result in a divide-by-zero error during the division of the signed operands num1
and num2
.
This code can result in a divide-by-zero error during the division of the signed operands num1
and num2
.
long num1, num2, result; /* Initialize num1 and num2 */ result = num1 / num2;
Compliant Solution (Division)
This compliant solution tests the divisor to guarantee there is no possibility of divide-by-zero errors.
long num1, num2, result; /* Initialize num1 and num2 */ if (num2 == 0) { // handle error } else { result = num1 / num2; }
Noncompliant Code Example (Remainder)
The %
operator provides the remainder when two operands of integer type are divided. This noncompliant code example can result in a divide-by-zero error during the remainder operation on the signed operands num1
and num2
.
long num1, num2, result; /* Initialize num1 and num2 */ result = num1 % num2;
Compliant Solution (Remainder)
This compliant solution tests the divisor to guarantee there is no possibility of a divide-by-zero error.
long num1, num2, result; /* Initialize num1 and num2 */ if (num2 == 0) { // handle error } else { result = num1 % num2; }
Risk Assessment
A division or remainder by zero can result in abnormal program termination and denial of service (DoS).
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|---|---|---|---|---|
NUM02-J | low | likely | medium | P6 | L2 |
Automated Detection
Automated detection exists for C and C++ but not for Java yet.
Related Guidelines
Bibliography
Section 6.5.5, Multiplicative Operators | |
Chapter 5, Integers | |
Chapter 2, Basics |