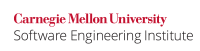
Methods return values to communicate failure or success and, at other times, to update the caller's objects or fields. Security risks can arise when method return values are ignored or when the invoking method fails to take suitable action on its receipt. When getter methods are named after an action, such as ProcessBuilder.redirectErrorStream()
, a programmer could fail to realize that a return value is expected. Note that the only purpose of the redirectErrorStream()
method is report via its return value whether the process builder merges standard error and standard output; the method that actually performs redirection of the error stream is the overloaded single-argument version. It is important to read the API documentation so that return values are not ignored.
Another example is ignoring the return value from add() on a HashSet. If duplicate, false will be returned.
Noncompliant Code Example (File Deletion)
This noncompliant code example attempts to delete a file, but fails to check whether the operation has succeeded.
File someFile = new File("someFileName.txt"); // do something with someFile someFile.delete();
Compliant Solution
This compliant solution checks the (boolean
) value returned by the delete()
method and handles errors as appropriate.
File someFile = new File("someFileName.txt"); // do something with someFile if (!someFile.delete()) { // handle failure to delete the file }
Noncompliant Code Example (String Replacement)
This noncompliant code example ignores the return value of the String.replace
method, failing to update the original string. The String.replace()
method cannot modify the state of the String
(because String
objects are immutable); rather, it returns a reference to a new String
object containing the desired result.
public class Ignore { public static void main(String[] args) { String original = "insecure"; original.replace( 'i', '9' ); System.out.println(original); } }
Compliant Solution
This compliant solution correctly updates the String
reference original
with the return value from the String.replace
method.
public class DoNotIgnore { public static void main(String[] args) { String original = "insecure"; original = original.replace( 'i', '9' ); System.out.println(original); } }
See also guideline [FIO02-J. Keep track of bytes read and account for character encoding while reading data].
Risk Assessment
Ignoring method return values can lead to unanticipated program behavior.
Guideline |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
EXP00-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
The Coverity Prevent Version 5.0 CHECKED_RETURN checker can detect the instance where Value returned from a function is not checked for errors before being used.
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this guideline on the CERT website.
Related Guidelines
C Secure Coding Standard: [seccode:EXP12-C. Do not ignore values returned by functions]
C++ Secure Coding Standard: [cplusplus:EXP12-CPP. Do not ignore values returned by functions or methods]
Related Guidelines
MITRE CWE: CWE-252
"Unchecked Return Value"
Bibliography
[[API 2006]] method delete() and method replace()
[[Green 2008]] "String.replace"
[[Pugh 2009]] misusing putIfAbsent
[!The CERT Oracle Secure Coding Standard for Java^button_arrow_left.png!] [!The CERT Oracle Secure Coding Standard for Java^button_arrow_up.png!] [!The CERT Oracle Secure Coding Standard for Java^button_arrow_right.png!]