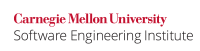
A nested class is any class whose declaration occurs within the body of another class or interface [[JLS 2005]]. The use of a nested class is error-prone unless the semantics are well understood. A common notion is that only the outer class can access the contents of the nested class. Not only does the nested class have access to the private fields of the outer class, the same fields can be accessed by another class within the package depending on whether the nested class is declared public or if it contains public methods or constructors. By default, the javac
compiler converts the accessibility of private methods of a nested class to package-private.
Also, according to the Java Language Specification [[JLS 2005]], §8.3 "Field Declarations,"
Note that a
private
field of a superclass might be accessible to a subclass (for example, if both classes are members of the same class). Nevertheless, aprivate
field is never inherited by a subclass.
Noncompliant Code Example
This noncompliant code example exposes the sensitive (x,y)
coordinates through the getPoint()
method of the inner class. Consequently, the AnotherClass
class that belongs to the same package can access the coordinates.
class Coordinates { private int x; private int y; public class Point { public void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); p.getPoint(); } }
Compliant Solution
Use the private
access specifier for hiding the inner class and all contained methods and constructors.
class Coordinates { private int x; private int y; private class Point { private void getPoint() { System.out.println("(" + x + "," + y + ")"); } } } class AnotherClass { public static void main(String[] args) { Coordinates c = new Coordinates(); Coordinates.Point p = c.new Point(); // fails to compile p.getPoint(); } }
Compilation of AnotherClass
now results in a compilation error because the class attempts to access a private nested class.
Risk Assessment
The Java language system weakens the accessibility of sensitive, private
entities in inner classes, which can result in a security weakness.
Recommendation |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
OBJ13-J |
medium |
probable |
medium |
P8 |
L2 |
Automated Detection
Automated detection of non-private nested classes that define non-private members and constructors is straightforward. However, this rule applies only when those classes could potentially expose sensitive data or operations from the outer class. Detection of sensitive data or operations requires programmer assistance.
Related Guidelines
Bibliography
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="c6431569-3bbb-4889-a39c-36af2945aae4"><ac:plain-text-body><![CDATA[ |
[[JLS 2005 |
AA. Bibliography#JLS 05]] |
[§8.1.3, Inner Classes and Enclosing Instances |
http://java.sun.com/docs/books/jls/third_edition/html/classes.html#8.1.3] ]]></ac:plain-text-body></ac:structured-macro> |
|
§8.3 "Field Declarations" |
|||
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="9d884b16-8f25-42a4-8b89-3ad419448b7b"><ac:plain-text-body><![CDATA[ |
[[Long 2005 |
AA. Bibliography#Long 05]] |
§2.3, Inner Classes |
]]></ac:plain-text-body></ac:structured-macro> |
<ac:structured-macro ac:name="unmigrated-wiki-markup" ac:schema-version="1" ac:macro-id="c151471d-1cae-4bcc-bbf6-89be2b68084d"><ac:plain-text-body><![CDATA[ |
[[McGraw 1999 |
AA. Bibliography#McGraw 99]] |
Securing Java, Getting Down to Business with Mobile Code |
]]></ac:plain-text-body></ac:structured-macro> |
OBJ08-J. Do not leak references to inner class objects when the outer class object maintains sensitive data 04. Object Orientation (OBJ)