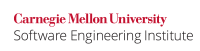
Mutable classes are those which when instantiated, provide a reference such that the contents of the class can be altered anytime. It is important to provide means for creating copies of mutable class instances so as to allow safe passing in and returning of class objects when used within method arguments.
Noncompliant Code Example
In this noncompliant example, MutableClass
uses a mutable Date
object. If the caller changes the instance of the Date
object (like incrementing the month), the class implementation no longer remains consistent with its old state. Both, the constructor as well as the getDate
method are susceptible to abuse. This also defies attempts to implement thread safety.
import java.util.Date; public final class MutableClass { private Date d; public MutableClass(Date d) { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } this.d = d; } public Date getDate() { return this.d; } }
Compliant Solution
Always provide mechanisms to create copies of the instances of a mutable class. This compliant solution implements the Cloneable
interface and overrides the clone
method to create a deep copy of both the object and the mutable Date
object. Since using clone()
independently only produces a shallow copy and still leaves the class mutable, it is advised to also copy all the referenced mutable objects that are passed in or returned from any method.
import java.util.Date; public final class CloneClass implements Cloneable { private Date d; public CloneClass(Date d) { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } this.d = new Date(d.getTime()); //copy-in } public Object clone() throws CloneNotSupportedException { SecurityManager sm = System.getSecurityManager(); if (sm != null) { //check permissions } final CloneClass cloned = (CloneClass)super.clone(); cloned.d = (Date)this.d.clone(); //copy mutable Date object return cloned; } public Date getDate() { return new Date(this.d.getTime()); //copy and return } }
At times, a class is labeled final
with no accessible copy methods. Callers can then obtain an instance of the class, create a new instance with the original state and subsequently proceed to use it. Similarly, mutable objects obtained must also be copied when necessary.
Risk Assessment
Creating a mutable class without a clone
method may result in the data of the class becoming corrupted.
Rule |
Severity |
Likelihood |
Remediation Cost |
Priority |
Level |
---|---|---|---|---|---|
SEC35-J |
low |
unlikely |
medium |
P2 |
L3 |
Automated Detection
TODO
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
[[API 06]] method clone()
[[Security 06]]
[[SCG 07]] Guideline 2-2 Support copy functionality for a mutable class
[[Bloch 08]] Item 39: Make defensive copies when needed
SEC34-J. Do not allow the unauthorized construction of sensitive classes 00. Security (SEC) SEC36-J. Ensure that the bytecode verifier is applied to all involved code upon any modification