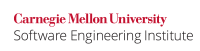
Holding locks while performing time-consuming or blocking operations can severely degrade system performance and can result in starvation. Furthermore, deadlock may can result if too many interdependent threads block indefinitely. Blocking operations include network, file, and console I/O (for example, invoking a method such as Console.readLine()
) , and object serialization. Deferring a thread indefinitely also constitutes a blocking operation. Consequently, programs must not perform blocking operations while holding a lock.
When the Java Virtual Machine If the Java Virtual Machine (JVM) interacts with a file system that operates over an unreliable network, file I/O might incur a large performance penalty. In such cases, avoid file I/O over the network when while holding a lock. File operations (such as logging) that may could block while waiting for the output stream lock or for I/O to complete may could be performed in a dedicated thread to speed up task processing. Logging requests can be added to a queue given , assuming that the queue's {{ Wiki Markup put()
}} operation incurs little overhead as compared to file I/O \ [[Goetz 06, pg 244|AA. Java References#Goetz 06]\Goetz 2006].
Noncompliant Code Example (
...
Deferring a
...
Thread)
This noncompliant code example defines a utility method that accepts a time
parameter. argument:
Code Block | ||
---|---|---|
| ||
public synchronized void doSomething(long time) throws InterruptedException { // ... Thread.sleep(time); } |
Because the method is synchronized, if when the thread is suspended, other threads are unable to cannot use the synchronized methods of the class. The current object's monitor is not released continues to be held because the Thread.sleep()
method does not have any synchronization semantics, as detailed in CON16-J. Do not assume that the sleep(), yield() or getState() methods provide lacks synchronization semantics.
Compliant Solution (
...
Intrinsic Lock)
This compliant solution defines the doSomething()
method with a timeout
parameter instead of rather than the time
value. The use of the Using Object.wait()
method instead of Thread.sleep()
allows setting a time out for a timeout period during which a notification may awaken the thread.
Code Block | ||
---|---|---|
| ||
public synchronized void doSomething(long timeout) throws InterruptedException { // ... while (<condition does not hold>) { wait(timeout); // Immediately leavesreleases the current monitor } } |
The current object's monitor is immediately released upon entering the wait state. After When the time out timeout period has elapsedelapses, the thread attempts to reacquire resumes execution after reacquiring the current object's monitor and resumes execution when it succeeds.
According to the Java API class {{Object}} documentation \[[API 06|AA. Java References#API 06]\]: Class Wiki Markup Object
documentation [API 2014]
Note that the
wait
method, as it places the current thread into the wait set for this object, unlocks only this object; any other objects on which the current thread may be synchronized remain locked while the thread waits.This method should only be called by a thread that is the owner of this object's monitor.
Ensure that a thread that holds Programs must ensure that threads that hold locks on other objects releases them release those locks appropriately , before entering the wait state. Also, refer to the related guidelines CON18Additional guidance on waiting and notification is available in THI03-J. Always invoke wait() and await() methods inside a loop and CON19THI02-J. Notify all waiting threads instead of rather than a single thread.
Noncompliant Code Example (Network I/O)
This noncompliant code example shows the method defines a sendPage()
method that sends a Page
object from a server to a client. The method is synchronized so that to protect the pageBuff
array pageBuff
is accessed safely when multiple threads request concurrent access.
Code Block | ||
---|---|---|
| ||
// Class Page is defined separately. // It stores and returns the Page name via getName() Page[] pageBuff = new Page[MAX_PAGE_SIZE]; public synchronized boolean sendPage(Socket socket, String pageName) throws IOException { // Get the output stream to write the Page to ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream()); // Find the Page requested by the client // (this operation requires synchronization) Page targetPage = null; for (Page p : pageBuff) { if (p.getName().compareTo(pageName) == 0) { targetPage = p; } } // Requested Page does not exist if (targetPage == null) { return false; } // Send the Page to the client // (does not require any synchronization) out.writeObject(targetPage); out.flush(); out.close(); return true; } |
Calling writeObject()
within the synchronized sendPage()
method can result in delays and deadlock-like conditions in high-latency networks or when network connections are inherently lossy.
Compliant Solution
This compliant solution entails separating separates the actions process into a sequence of steps:
- Perform actions on data structures requiring synchronization.
- Create copies of the objects
...
- to be sent.
- Perform network calls in a separate
...
- unsynchronized method.
In this compliant solution, the unsynchronized sendPage()
method calls the synchronized method getPage()
is called from an unsynchronized method sendPage()
, to find retrieve the requested Page
in the pageBuff
array. After the Page
is retrieved, the method sendPage()
calls the unsynchronized method deliverPage()
method to deliver the Page
to the client.
Code Block | ||
---|---|---|
| ||
// No synchronization public boolean sendPage(Socket socket, String pageName) { // No synchronization Page targetPage = getPage(pageName); if (targetPage == null){ return false; } return deliverPage(socket, targetPage); } // Requires synchronization private synchronized Page getPage(String pageName) { // Requires synchronization Page targetPage = null; for (Page p : pageBuff) { if (p.getName().equals(pageName)) { targetPage = p; } } return targetPage; } // Return false if an error occurs, true if successful public boolean deliverPage(Socket socket, Page page) { ObjectOutputStream out = null; boolean result = true; try { // Get the output stream to write the Page to ObjectOutputStream out = new ObjectOutputStream(socket.getOutputStream()); // Send the Pagepage to the client out.writeObject(page);out.flush(); } catch (IOException io) { // If recovery is not possible return false return false; } finally { out.flushresult = false; } finally { if (out != null) { try { out.close(); out.close(); } catch (IOException e) { result = false; } } } return trueresult; } |
Exceptions
EX1LCK09-J-EX0: Classes that are compliant with the guideline CON24provide an appropriate termination mechanism to callers are permitted to violate this rule (see THI04-J. Ensure that threads and tasks performing blocking operations can be terminated in that they provide an appropriate termination mechanism to callers, are allowed to violate this guideline.).
LCK09-J-EX1: Methods that require EX2: A method that requires multiple locks may hold several locks while waiting for the remaining locks to become available. This constitutes a valid exception, though care must be taken to avoid deadlock. See CON12although the programmer must follow other applicable rules, especially LCK07-J. Avoid deadlock by requesting and releasing locks in the same order for more information to avoid deadlock .
Risk Assessment
If blocking Blocking or time consuming lengthy operations are performed within synchronized regions , temporary or permanent deadlock may resultcould result in a deadlocked or unresponsive system.
Rule | Severity | Likelihood | Remediation Cost | Priority | Level |
---|
LCK09-J |
Low |
Probable |
High | P2 | L3 |
Related Vulnerabilities
Search for vulnerabilities resulting from the violation of this rule on the CERT website.
References
Wiki Markup |
---|
\[[API 06|AA. Java References#API 06]\] Class {{Object}}
\[[Grosso 01|AA. Java References#Grosso 01]\] [Chapter 10: Serialization|http://oreilly.com/catalog/javarmi/chapter/ch10.html]
\[[JLS 05|AA. Java References#JLS 05]\] [Chapter 17, Threads and Locks|http://java.sun.com/docs/books/jls/third_edition/html/memory.html]
\[[Rotem 08|AA. Java References#Rotem 08]\] [Falacies of Distributed Computing Explained|http://www.rgoarchitects.com/Files/fallacies.pdf] |
Automated Detection
Some static analysis tools are capable of detecting violations of this rule.
Tool | Version | Checker | Description | ||||||
---|---|---|---|---|---|---|---|---|---|
CodeSonar |
| JAVA.CONCURRENCY.STARVE.BLOCKING | Blocking in Critical Section (Java) | ||||||
Parasoft Jtest |
| CERT.LCK09.TSHL CERT.LCK09.TSHL2 | Do not use blocking methods while holding a lock Do not call 'Thread.sleep()' while holding a lock since doing so can cause poor performance and deadlocks | ||||||
PVS-Studio |
| V6095 | |||||||
ThreadSafe |
| CCE_LK_LOCKED_BLOCKING_CALLS | Implemented | ||||||
SonarQube |
| S2276 | Implemented |
Related Guidelines
Bibliography
...
CON19-J. Notify all waiting threads instead of a single thread 11. Concurrency (CON) CON21-J. Use thread pools to enable graceful degradation of service during traffic bursts